JS Plugin
The Javascript (JS) Plugin is the recommended way to add Vouched to your web application.
NEW - JS Plugin Wizard
Try out our new JS Plugin Wizard here.
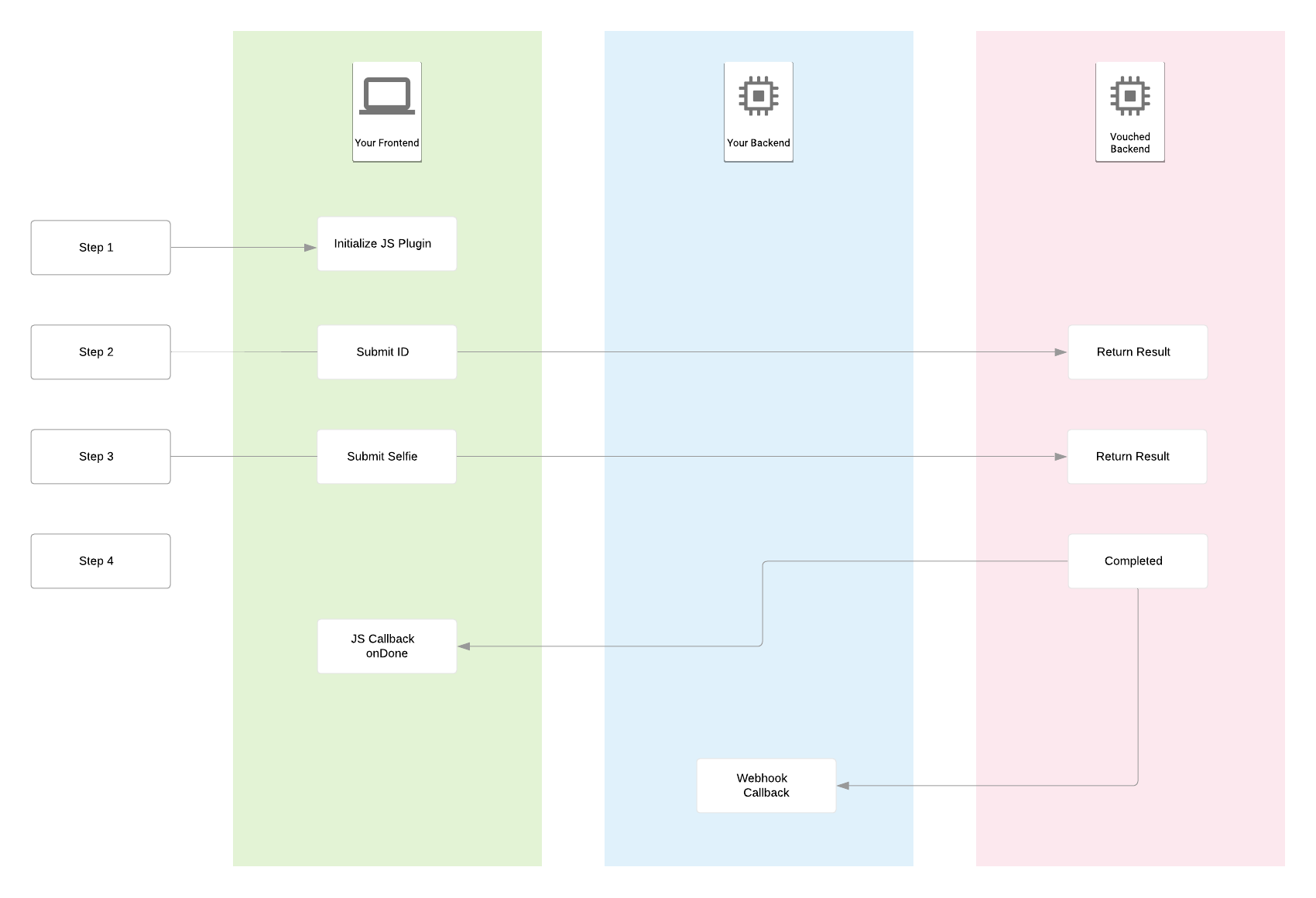
JS Plugin Flow
- Create your public key
- Copy the Quick Start Code below or follow the recipe to add Vouched to your site
Camera Restrictions
Browser permissions for the camera require an
https
host. Sites hosted withhttp
orfile://
will not work. For testing purposes, alocalhost
site is a viable option.
Quick start code
Vouched
Lifetime considerationsThe
Vouched
function and subsequent calls to.mount()
should only happen once per verification session otherwise multiple instances of the plugins which is not supported and may lead to severe issues.
<!DOCTYPE html>
<html>
<head>
<!-- utf-8 is required for JS Plugin default fonts -->
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<script src="https://static.vouched.id/plugin/releases/latest/index.js"></script>
<script type='text/javascript'>
(function() {
var vouched = Vouched({
// Optional verification properties.
verification: {
// verify the user's information
firstName: 'Gladys',
lastName: 'West',
// used for the crosscheck feature
email: '[email protected]',
phone: '000-111-2222'
},
liveness: 'straight',
appId: '<PUBLIC_KEY>',
// your webhook for POST verification processing
callbackURL: 'https://website.com/webhook',
// mobile handoff
crossDevice: true,
crossDeviceQRCode: true,
crossDeviceSMS: true,
// called when the verification is completed.
onDone: (job) => {
// token used to query jobs
console.log("Scanning complete", { token: job.token });
// An alternative way to update your system based on the
// results of the job. Your backend could perform the following:
// 1. query jobs with the token
// 2. store relevant job information such as the id and
// success property into the user's profile
fetch(`/yourapi/idv?job_token=${job.token}`);
// Redirect to the next page based on the job success
if( job.result.success){
window.location.replace("https://website.com/success/");
}else{
window.location.replace("https://website.com/failed/");
}
},
// theme
theme: {
name: 'avant',
},
});
vouched.mount("#vouched-element");
})();
</script>
</head>
<body>
<div id='vouched-element' style="height: 100%"/>
</body>
</html>
Basic react example
import React, { useEffect, useRef } from 'react';
/**
* This component will mount the `Vouched` verification plugin and unmount it when the component is no longer rendered.
*/
const VouchedComponent = () => {
const id = 'vouched-container';
const vouchedRef = useRef();
// You must ensure the `Vouched` function is only called once per verification session.
useEffect(() => {
// Assuming vouched is included via script tag or otherwise loaded
if (!window.Vouched || vouchedRef.current) {
return;
}
const instance = window.Vouched({
// your configuration
});
vouchedRef.current = instance;
instance
.mount(`#${id}`)
.then(() => {
// optionally await mounting, usually not required when using callbacks.
console.log('vouched mounted');
});
return () => {
instance.unmount(`#${id}`);
};
}, []);
return <div id={id} />;
};
Versioning considerations
Note about legacy versioning scheme
For historic & compatibility reasons the plugin located at
https://static.vouched.id/widget/vouched-2.0.0.js
is available until we announce its deprecation.
Future npm package
We are currently working on publishing a version of our plugin via npm, we expect to ship this with
v3
of the plugin.This will allow you to leverage tools such as Dependabot to make automatic updates.
The vouched plugin is released according to semantic versioning.
We generally recommend embedding the latest
version of plugin as we make frequent fixes and improvements to our plugin.
However while we try to keep breaking changes at a minimum certain changes may negatively impact when automatically adopting major
version changes.
In order to restrict automatic embedding of future versions you can pin the version of the plugin you wish to use to major
,minor
or specific version.
Example
- Always using the latest version
https://static.vouched.id/plugin/releases/latest/index.js
- Pinning
major
version onlyhttps://static.vouched.id/plugin/releases/v2/index.js
- Pinning
major
andminor
https://static.vouched.id/plugin/releases/v2.0/index.js
- Pinning to exact
patch
versionhttps://static.vouched.id/plugin/releases/v2.0.0/index.js
A note on Sub Resource Integrity
SRI is only supported when using fully qualified versions since the contents of the files served under not fully qualified are mutable.
Examples
latest
is not fully qualifiedv3
andv3.2
are not fully qualified since the minor and patch version may implicitly change.v3.2.1
is fully qualified
In order to use SRI we suggest following this guide.
Layout Considerations
When integrating Vouched into your site design, consider that the Vouched component occupies 100% height of the parent container (it has CSS rule height: 100%
applied). That means the parent container should be given a height.
Height should be given, for example, by setting height
value (height: 100%
) on the container or by using flex constraints (flex-grow: 1
in vertical flex-box
).
On a desktop device, you will see different results depending on your crossDevice
, crossDeviceQRCode
, and crossDeviceSMS
values. If they are all true as shown in the above code example, you can read more details on our Mobile Handoff page. If these fields are not present or set to false, you will see the following:
Javascript Callbacks
A callback is a function that is to be executed after another function has finished executing β hence the name "call back". Functions can take other functions as arguments. Functions that do this are called higher-order functions. Any function that is passed as an argument is called a callback function.
In the context of Vouched, the callback functions can be used to send data back to your server or interact with your app as desired. Some examples are onSubmit
(this function is called when a user submits a photo), onCamera
(this function is called when camera initialization is complete), onInit
(called during initialization of the Web App), and onDone
(called when the verification is complete). Most of these provide access to the job
object of type Job
, which contains useful information such as job.id
, job.token
, job.result.success
, and job.status
.
Arguments
Parameters | Type | Description |
---|---|---|
appId | string | Public key (Required). |
type | string | Default: idv Enums: idv , id , reverify The job type. idv - ID with selfie verification id - ID only reverify - reverification |
token | string | Time-limited session token used to restart a session. Tokens are valid for 15 days. |
crossDevice | boolean | Default: false Provides the ability to maintain the same session across multiple devices using the token retrieved from onInit |
crossDeviceQRCode | boolean | Default: false If the user is on a desktop computer, a scannable QR Code enables the verification to continue on their mobile phone. Once completed, control is given back to the desktop. |
crossDeviceSMS | boolean | Default: false If the user is on a desktop computer, the user can input a cell phone number and we will text a secure link to continue verification. |
crossDeviceURL | string | Option to set a custom domain for cross device handoff. Contact [email protected] to enable this feature. |
showUploadFirst | boolean | Default: true Show an instructions screen before the camera selfie steps capture. |
face | string | Default: camera Enums: upload , camera , both Specify how user can submit their selfie. |
id | string | Default: both Enums: upload , camera , both Specify how user can submit their id. |
idLiveness | string | Default: null ID Card liveness check. Add distance to activate liveness check. idLiveness checks for user movements with ID Card. |
liveness | string | Default: mouth Enums: mouth , orientation , distance , straight Only applicable for selfie/headshot liveness check. Specify how Vouched should check for face liveness. The mouth liveness, checks for mouth movements, orientation checks the facial orientation, distance checks for user movements, and straight checks if the user is looking at the camera. |
enableEyeCheck | boolean | Default: false Only applicable for selfies. Checks if user kept their eyes open before selfie image is captured. |
properties | array | Arbitrary properties to add to the job. Array of objects with name and value parameters.Example: [ {name: "Foo", value: "Bar"}, {name: "Baz", value: "Qux"} ] |
content | object | Properties to change the text content during the verification process. |
verification | object | Optional verification properties. |
callbackURL | string | Upon the job's completion, Vouched will POST the job results to this URL. See webhooks for more info. |
stepTitles | object | Titles for steps of the verification process. |
theme | object | Change the theme and styles of the plugin. |
includeBarcode | boolean | Default: false Enable ID barcode capture and processing for applicable IDs. The error BarcodeMatchError results when idMatch falls below the normalized threshold or the user skips the barcode step. |
includeBackId | boolean | Default: false Enables capture for the back of the ID for all jobs. |
disableCssBaseline | boolean | Default: false Disables the internal CSS baseline styles |
onSubmit | function:(props: { stage: string; attempts: number; job: Job; }): void Job | Javascript callback when a user submits a photo |
onCamera | function:(props: { hasCamera: boolean; hasPermission: boolean; }): void | Javascript callback when camera initialization is complete |
onCameraEvent | function:(cameraEvent: CameraEvent): void CameraEvent: { cameraRendered?: boolean; }` | Javascript callback when there are changes to the Camera DOM element. |
onInit | function:(props: { token: string; job: Job; }): void Job | Javascript callback during initialization of the web app |
onDone | function:(job: Job): void Job | Javascript callback when a verification is complete |
onReverify | function:(job: Job): void Job | Javascript callback when a reverification job is complete |
onConfirm | function:(userConfirmEvent: UserConfirmEvent): void UserConfirmEvent : { confirmedExtractedData?: boolean; } | Javascript callback after user confirmation. See userConfirmation |
onSurveyDone | function(job: Job): void Job | Javascript callback when a survey is complete. Contact [email protected] |
showProgressBar | boolean | Default: true Flag to show the Progress Bar (Note this configuration is available for Avant theme ) |
maxRetriesBeforeNext | number | Default: 0 Number of times to force users to retry before showing the Next button (Default: 0 - show Next button immediately). Deprecated alias: idShowNext |
numForceRetries | number | Default: 2 Number of times to force users to retry before showing the Next button in force-retry cases (the card doesn't meet the minimum threshold). |
handoffView | object | Settings for displaying the QR code when Mobile Handoff is enabled. |
locale | string | Default: en Enums: en , es , fr , fr_CA Specify the language (English, Spanish, French, or French Canadian). |
enableGeoLocation | boolean | Default: false Enables GeoLocation for the user and returns the user's longitude and latitude (Returns error message indicating if user has denied sharing their location or browser/device does not have location support). |
userConfirmation | object | Properties used for the user confirmation feature. |
manualCaptureTimeout | number | Default: 12000 Number (in ms) to specify the time it takes for the manual capture button to show up. |
content Object
Optional properties to change the text content during the verification process.
Property | Type | Description |
---|---|---|
success | string | The messages posted after successful submission. Example: 'Please close this window to return your online visit.' |
review | string | Response to the user describing review of results. Example: 'Thank you for providing your information. We will review and get back to you.' . |
crossDeviceSuccess | string | Message posted after the crossDevice verification success. Example: 'Verification is complete, continue on your desktop.' . |
crossDeviceInstructions | string | Instructions and requirements for crossDevice. Example: 'We need to verify your identity. This requires government-issued photo ID as well as selfie. Please follow the instructions below to continue the verification process on your phone:' 1. Open the Camera App from your phone . 2. Hold the device so the QR Code appears in the viewfinder . 3. Click on notification to open the verification link . |
crossDeviceTitle | string | Title for crossDevice. Example: 'Identity Verification' . |
crossDeviceShowOff | boolean | Allows Handoff to continue on Desktop. |
upperStartInstructions | string | Upper Instructions on Start Screen. |
middleStartInstructions | string | Middle Instructions on Start Screen. |
upperIdInstructions | string | Upper Instructions on ID Screen. |
lowerIdInstructions | string | Lower Instructions on ID Screen. Example: "You can either upload a photo you've already taken, or take a photo of your ID now." . |
upperBackIdInstructions | string | Upper Instructions on Back ID Screen. |
lowerBackIdInstructions | string | Lower Instructions on Back ID Screen. |
upperFaceInstructions | string | Upper Instructions on Face Screen. |
lowerFaceInstructions | string | Lower Instructions on Face Screen. |
lowestFaceInstructions | string | Lowest Instructions on Face Screen. |
upperIdCapturedInstructions | string | Upper Instructions on ID Captured Screen. |
middleIdCapturedInstructions | string | Middle Instructions on ID Captured Screen. |
middleBackIdCapturedInstructions | string | Middle instructions on ID Captured Screen when Barcode scan is enabled. |
lowerIdCapturedInstructions | string | Lower Instructions on ID Captured Screen. |
cameraIDButton | string | String text for camera button on ID Screen. |
cameraButtonLabelUploadId | string | String text for Upload button on ID Screen. |
cameraFaceButton | string | String text for camera button on Face Screen. |
cameraButtonLabelUploadFace | string | String text for Upload button on Face Screen. |
upperSuccess | string | Success message at the top. Example: 'Your photo uploads are complete!' . |
lowerSuccess | string | Success message at the bottom. Example: 'Thank you.' . |
upperFailure | string | Failure message at the top. Example: 'Try Again' . |
lowerFailure | string | Failure message at the bottom. Example: "The photo you shared can't be used for validation. Please take another picture, making sure the image of your face or your ID is clear." . |
verifyPass | string | The verification passed. Example: 'Everything looks good to us. Check your information and click next.' . |
verifyFail | string | The verification failed. Example: "We couldn't verify you. If you disagree, update your information and click next." . |
qrHandoffInstructions | string | Optional bottom Handoff Instructions. |
qrDesktopInstructions | string | Instructions for crossDeviceShowoff. qrDesktopInstructions is the instructions and requires '{qrDesktopLink}' to be set to inject text for the link. Default: 'Alternatively, you can {qrDesktopLink} if you have a good desktop camera.' . |
qrDesktopLink | string | String text used in qrDesktopInstructions to replace {qrDesktopLink} . qrDesktopLink becomes the link text. Example: Continuing the example above, if qrDesktopLink = 'continue', qrDesktopInstructions becomes 'Alternatively, you can continue if you have a good desktop camera.' . |
startCompanyInstructions | string | Optional Start Screen Instruction. |
carouselCompanyText | string[] | Array of strings where string at each index make up the Carousel Slide. |
carouselCompanyImg | string[] | Array of strings (image URL) where image at each index make up the Carousel Slide. |
progressIndicatorLoading | string | String that would replace term 'loading' in the Progress Indicator. |
progressIndicatorVouching | string | String that would replace term 'vouching' in the Progress Indicator. |
upperProgressIndicatorId | string | String that would replace the upper text on ID processing progress screen on Avant theme |
lowerProgressIndicatorId | string | String that would replace the lower text on ID processing progress screen on Avant theme |
upperProgressIndicatorFace | string | String that would replace the upper text on Selfie processing progress screen on Avant theme |
lowerProgressIndicatorFace | string | String that would replace the lower text on Selfie processing progress screen on Avant theme |
startScreenButtonLabel | string | Optional string to override the button text in the Avant Start screen. |
cameraTopIDDirections | string | Optional string to override the top text in the Avant ID Camera screen. |
cameraTopBackIdDirections | string | Optional string to override the top text in the Avant Back ID Camera screen |
cameraTopFaceDirections | string | Optional string to override the top text in the Avant Face Camera screen. |
cameraButtonLabelContinueSelfie | string | Optional string to override the button text in the Avant ID Captured screen. |
retryMessageInvalidIdPhoto | string | Default: We could not recognize the ID Optional prop to override the first line of the retry message in the ID screen. |
retryMessageInvalidUserPhoto | string | Default: We could not recognize the selfie Optional prop to override the first line of the retry message in the Face screen. |
cameraScreenLabelFrontId | string | Optional prop to override the initial camera labels in the FrontID Camera Screen. |
cameraScreenLabelBackId | string | Optional prop to override the initial camera labels in the BackID Camera Screen. |
cameraFaceLabelShowFace | string | Optional prop to override the initial camera labels in the Face Camera Screen. |
mobileHandoffDoneMessage | string | Optional prop for extra text on the Done Screen when doing Handoff on Mobile. |
surveyButtonLabel | string | String text for submit button on Survey Screen. |
verification Object
Optional verification properties.
Property | Type | Description |
---|---|---|
firstName | string | Used to compare to the first name extracted from the ID document during Identity Verification. Example: Jerry |
lastName | string | Used to compare to the last name extracted from the ID document during Identity Verification. Example: Lawson |
birthDate | string | Used to compare to the date of birth extracted from the ID document during Identity Verification. Example: 12/01/1940 |
email | string | Used for identity Crosscheck. |
phone | string | Used for identity Crosscheck. |
enableIPAddress * | boolean | Enable IP address check. *This is a paid feature, reach out to [email protected] before turning on |
enablePhysicalAddress * | boolean | Enable physical address check. *This is a paid feature, reach out to [email protected] before turning on |
enableCrossCheck * | boolean | Enable phone, email, and address Crosscheck. *This is a paid feature, reach out to [email protected] before turning on |
enableDriversLicenseValidation * | boolean | Enable Driver License Verification check on the ID. * This is a paid feature, reach out to [email protected] before turning on |
stepTitles Object
Titles for steps of the verification process.
Property | Type | Description |
---|---|---|
FrontId Required | string | The message shown on the ID screen. Example: "Front ID" . |
Face Required | string | The message shown on the face screen. Example: "Face" . |
Done Required | string | The message shown on the completion screen. Example: "Done verifying" . |
theme Object
Property | Type | Description |
---|---|---|
name | string | Specifies the JS Plugin theme to use. Default: verbose Enums: verbose , avant , classic (deprecated) |
iconLabelColor | string | Hex value for Icon Label color. Compatible with theme: verbose Example: '#413d3a' . |
bgColor | string | Hex value for Background color. Compatible with theme: verbose Example: '#FFFFFF' . |
logo | object | Logo. Compatible with theme: verbose , avant Example: logo: { src: 'https://www.vouched.id/wp-content/uploads/2020/11/vouched_logo_hi_res.png ', style: { 'max-width': 150, 'margin-bottom': 30 }} |
navigationActiveText | string | Hex value for navigation active text. Compatible with theme: verbose Example: '#413d3a' . |
iconColor | string | Hex value for Icon color. Compatible with theme: verbose Example: '#f6f5f3' . |
iconBackground | string | Hex value for Icon Background color. Compatible with theme: verbose Example: '#c8ae8f' . |
baseColor | string | Hex value for primary color. Compatible with theme: verbose , avant Example: '#4d7279' . |
fontColor | string | Hex value for font color. Compatible with theme: verbose Example: '#413d3a' . |
font | string | Font Family. Compatible with theme: verbose , avant Example: 'Open Sans' . |
navigationActiveBackground | string | Hex value for background color of active breadcrumbs. Compatible with theme: verbose Example: '#bacbd1' . |
navigationDisabledBackground | string | Hex value for background color of disabled breadcrumbs. Compatible with theme: verbose Example: '#bacbd1' . |
navigationDisabledText | string | Hex value for text color on disabled breadcrumbs. Compatible with theme: verbose Example: '#4d7279' . |
secondaryButtonColor | string | RGB value for color of the secondary buttons (Grey Retry Next button). Default: 'rgb(216, 216, 216)' . |
handoffLinkColor | string | Hex value for text color in the Mobile Handoff verification link. Default: '#40a1ed' . |
progressIndicatorTextColor | string | Hex value for text color of the progressIndicator in Avant theme. Default: '#000' . |
handoffView Object
Settings for displaying the QR code when Mobile Handoff is enabled.
Property | Type | Description |
---|---|---|
qRCodeSize | number | Default: undefined The QR Code canvas element size in pixels. When undefined the size will be determined. |
onlyShowQRCode | boolean | Default: false Only shows the QR code. |
reverificationParameters Object
Settings for Reverification (if enabled).
Property | Type | Description |
---|---|---|
jobId | string | The ID of the completed source verification job to reverify against. |
match | string | Default: selfie Enums: id , selfie . Matches the captured image to either the source job's ID image or Selfie image. |
userConfirmation Object
Settings for user confirmation of photos (if enabled).
Property | Type | Description |
---|---|---|
confirmData | boolean | Shows the user the extracted data after ID photo is processed. |
confirmImages | boolean | Shows the user each photo taken before photo is processed. |
Unmount JS Plugin
vouched.unmount('#vouched-element')
Updated about 2 months ago